No pretty screenshots today. Just long walls of densely-packed text. Suck it up. We can’t work on bling-mapping every day.
I’ve mentioned that this was the big hurdle in my project. This is where I’ve been planning to run aground and give up. Two two or three times in the past I’ve tried to nail this down, only to get lost and frustrated, and eventually give up.
An animated character is a seriously complex beast. There are a lot of steps, all of them are hard, and a mistake in any of them will mangle the end product. The process goes something like this:
- Pick a 3D file format out of the dozens available. Collada files? 3DS Max files? Maya? .X? No file format is perfect. Some are harder to read than others. (And some are absolute devils to read correctly.) Some have acceptable documentation. Some are proprietary file formats that have been (partly) reverse-engineered by enterprising go-getters. Some have only partial or conflicting documentation for how to read them. There are a lot of different types of information that you will need, and a lot of information you won’t need. Surface normals, texture mapping coords, animation data, vertex weighting, surface materials & lighting properties. Some files include non-model stuff like the position of lights and the background you’re using in your 3D program. You need to find a format that is as easy to read as possible, has the data you need, and has as little extra stuff as possible.
- With your file format all picked out, you have to write an importer. Read that data into your program, convert it from its native coordinate system into the one used by your game, and turn it into polygons for rendering.
- Pick out an animation file format. This is a file that will describe the movements for your character. These poses will describe how to make your character walk, hold a gun, crouch behind chest-high walls, crouch over the face of fallen enemies, or whatever else your characters need to be able to do. (Although apparently those are the only things anyone cares about?) Choosing this file format is a lot like the process in step 1. (Some formats support both models and animations, but you still have to read in this other data. And using the same files for both comes with its own set of problems, which I’ll talk about later.)
- Write an importer for that animation data.
- Write a system to apply those animations to the model. You need to be able to smooth animation frames, blend between animations, play animations back at varying speeds, and a bunch of other stuff. The animations move the character’s skeleton. The skeleton moves the vertices, which move the polygons, which makes the magic happen.
- Now fire up your program. Doesn’t work, does it? The limbs are flailing around randomly. Is it a problem with your model importer? A problem with the animation importer? A problem translating from the foreign coordinate system to your own? A discrepancy preventing the two from properly relating to one another? Or perhaps your understanding of the animations, and both file formats is perfect, but you just have a plain old bug in your implementation? Congratulations. Have fun fishing around in those 2,000 lines of code, looking for a problem that you might not even be able to spot if I pointed to it. You are well and truly screwed.
Previously, this was how I tried to do it, which is why I failed. I’m taking a somewhat more cautious approach this time. This time, I’m going to work in the opposite direction, starting with the animation system and working my way backwards to importing models. Of course, I can’t very well develop an animation system without something to animate. So I make this guy:
![]() |
A stickman. (Or, given the rather lopsided ratio of legs to torso, perhaps this is a female? Bah. YOU do better by trying coords in a text editor.) He’s actually more a collection of points than a proper “stick” man. The lines you see are just there to show the relationship between the points. Each point draws a line to its parent. I made this thing by hand, using code. Kind of annoying and a slight time sink, but it guaranteed that my skeleton would be exactly as I intended, without needing to write an importer first or wonder if it was working properly.
The next step is to get him moving. I don’t have an animation system yet, so I’m just having him rotate his knee on a sine wave.
![]() |
Next, I stick a polygon to him. This is done by assigning the vertex points to a particular joint. (People think of skeletons as bones, but in graphics skeletons are really joints. You can see this when you look at models created by different artists. Some name their skeleton parts, upperarm, forearm, hand. Others would name those same parts shoulder, elbow, and wrist. Neither one is technically wrong, as long as everyone agrees on how things should be named.) Roughly, this process boils down to me saying, “This vertex belongs to the knee joint. When the knee bends, this vertex needs to pivot in space around the knee.”
It works. If I bend the knee, the polygon moves in space with it, making it look like the polygon is “attached” to the bone. If I move the hip, it rotates around the hip properly.
This should also explain the bug you may see in videogames once in a long while: You’ll see this brief glimpse of a character standing arms-out, feet slightly spread. That’s a character that hasn’t had any animation applied.
![]() |
I add a few more polygons, placing them right where his bones would be. I apply a bunch of sine waves to all the joints, and he begins bending and twisting in space. All the polygons go where they should. None drift off or pivot around the wrong point.
It’s stupid, ugly, and primitive, but the important thing is that it is correct. Now I can make an importer. When things go wrong (and they will go wrong) I’ll know it’s a problem with the importer, secure in the knowledge that the underlying animation stuff is correct.
The Plot-Driven Door
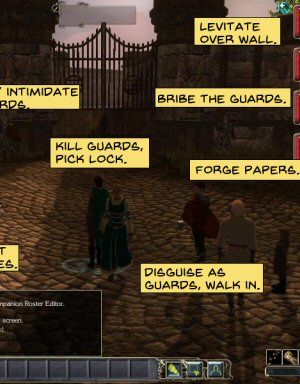
You know how videogames sometimes do that thing where it's preposterously hard to go through a simple door? This one is really bad.
Object-Oriented Debate
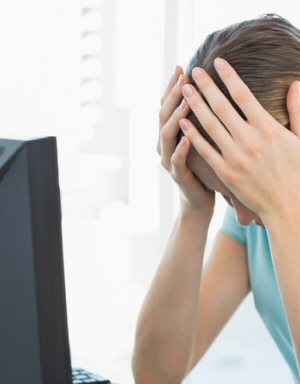
There are two major schools of thought about how you should write software. Here's what they are and why people argue about it.
How I Plan To Rule This Dumb Industry
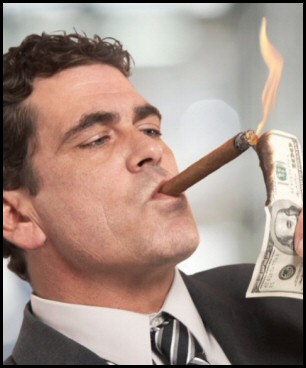
Here is how I'd conquer the game-publishing business. (Hint: NOT by copying EA, 2K, Activision, Take-Two, or Ubisoft.)
Playstation 3
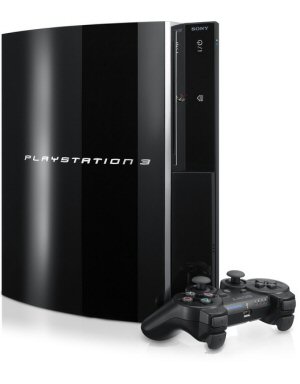
What was the problem with the Playstation 3 hardware and why did Sony build it that way?
Another PC Golden Age?
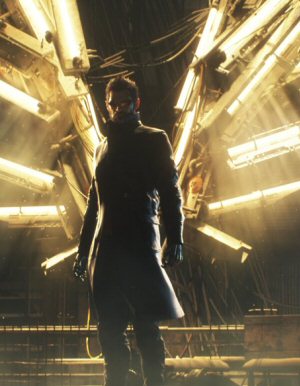
Is it real? Is PC gaming returning to its former glory? Sort of. It's complicated.
ouch. sounds like hard work. on the up side, sounds like hard work that makes a whole bunch of other things less painful.
reducing the number of potential failure points you have to deal with at one time seems like it would have difficulty being a bad plan :D
very interesting.
Loving the “Stick” Man–totally feeling it. In fact, I strongly believe this should be the next gen. game hero. He has a unique, unmistakable look to him, and it would be a nice change of pace from all the gruff, unshaven white male warriors. :D
As a matter of fact, I think that with some refinement this could me the next minecraft guy :-)
edit: I made another comment, but maybe went in spam or moderation cause I used 4 links. It’s pretty strict the law about linking outside, isn’t it?
I’ve had comments blocked as spam because they included one link, and tht was to the escapist, a website that Shamus regularly links to. I was linking to a LRR video recently, that I thought someone might have been referencing. This was an escapist address, yet it was blocked. The spam filter seems odd on this site sometimes.
Shamus, if this is a first person game, is it strictly necesarry to have character models at all, or am I in error in not considering multiplayer?
Yeah, the spam filter here is goofy sometimes. It’s supposedly tripped by 4 or more links, but often just 1 link can set it off. I don’t know why. If I’m lucky, it gets caught in moderation and I can restore it easily. If it goes all the way into the spam filter, I won’t know about it. The spam filter get too much crap for me to regularly sift through it.
You’re assuming he’s making a character model for the user to control. Maybe he just wants to populate his world with wandering stickmen.
Personally, I think it’d be neat if you’d make weird conical-looking animals to go with the conical trees, it’d give the place an interesting “alien” feel to it.
Well even if strictly single player, you will probably need NPCs, which obviously would use the same animation system.
I was under the impression that the game would be very solitary, with perhaps no humanoid NPCs, but this was only from my own ideas about the game from what I’ve seen so far. Some sort of animation system is necesarry, and in retrospect I was stupid to say that it might not be. What I originally meant was that other things might be higher priority, but Shamus has given reasons why he wants to do this now.
Reason for doing it now: If it’s going to kill the project, better sooner than later.
Thats not the way to do it. The trick is to get so far into the project, and put so many of your hours into it, that you feel intense shame for not finishing it.
One day a game powered only by shattered dreams and shame will be created.
Wasn’t Duke Nukem Forever released recently?
Bazinga!
…Yeah, I just snorted tea over my desk when reading that. Thank you, sir.
Now I need to clean my desk…
We all, including himself, know Shamus will quit and just feel mad at himself for a bit, and then go back to his book.
It’s okay Shamus, we understand
Are you assuming that everyone plays in first person? I always use third person perspectives because first person perspective makes me sick as a dog to watch it.
Edit: this was supposed to be a reply to zigzag.
That stickman has more character than a busful of generic RPG protagonists put together. I mean, he even has colors other than brown. I say, stick with him.
He does look surprisingly in his element in that last screenshot.
He kinda looks like he’s pointing out something.
‘And over there you can see our endless field of flowers… yeah, we’re pretty proud of that. Took a while to get right, I won’t mind tellin’ ya.’
I thought he was charging admission from off-screen stick people waiting in a queue to get into the pretty meadow. I can just hear him saying “Tickets please. Have your tickets ready as you step up…”
It’s just a model!
… seriously, people, this stick figure has just been photoshopped onto the background, and not very well either. No shadow, hard transition between figure and background, not even sure about the lighting …
The image is probably on a billboard somewhere in cube land.
“Come to arbitrary-polygon land! We have all sorts of angles, from slight inclinations to steep mountains. Come to where coordinates are more than just integers!”
Most people in cube land hate it, though, because they can’t deal with all of this “in-between-discrete-states” stuff. It’s probably a lie anyway. I mean, you’re either at 1 or at 2, right? What would be the point of being at 1,34582? That’s both useless and complicated, so go away, thank you.
… seriously, people, this stick figure has just been photoshopped onto the background, and not very well either. No shadow, hard transition between figure and background, not even sure about the lighting …
I’m not sure if that is sarcasm or not… I’m not sure if i’ve ever seen a serious lie from Shamus in any post. And so what that he hasn’t implemented the a shadow/lighting system on something he isn’t going to keep in the game and is just using to get an animation system working?
I think you shouldn’t take him seriously even though he says “seriously, people”.
But he said “seriously”!
I totally understand your frustration with animation Shamus, it one of the things that has scared me off from a making videogames of my own. I can’t really wrap my head around how complex it is. That being said, if I decided to work at a huge game developer, I could probably avoid this altogether and work on what I enjoy most (level design).
It’s actually easier to learn about character animation than you would think and bears similarities to level building. I did some character animation for a 2d game with a programmer’s tool and it worked like this from the ground up.
– you build an individual frame for a character out of sprites drawn by an artist and scanned in computer.
– you take the frames add them to an animation sequence as keyframes. -you use keyframes to store information needed for the animation, such as play duration, special effects, and scripts for playing sounds or transitioning to other animation sequences.
-you save you animation sequence and feed it into your game.
Still, that was for a 2d game, which is MUCH easier to work on than a 3d game. My hat’s off to you Seamus for building it from the ground up. Do you plan to use triggers or scripts for your animation engine?
I think the reason I don’t like animation is because I’m so piss poor bad at it.
Actually anything that involves rendering something even remotely resembling a human being is near impossible for me. Something about the human form is just over my head, which sucks, because I took human anatomy classes and labs in college. :/
Don’t know if you …well.. know , is another game that is going along the minecraft payment method, in which you buy, at a discounted price, the access to the weekly alphas. In their blog they illustrate the changes between every iteration and sometimes add few interesting side videos.
This post about the animation and character issue reminded me of these post: ; and .
These videos illustrate quite well what could possibly the issues with programming a charcter even to an unitiated like myself.
:-)
And because I missed a closing tag the comment got all messed up.
Let’s make this thing easier:
Don't know if you know Overgrowth, is another game that is going along the minecraft payment method, in which you buy, at a discounted price, the access to the weekly alphas. In their blog they illustrate the changes between every iteration and sometimes add few interesting side videos.
This post about the animation and character issue reminded me of these post:
1) http://blog.wolfire.com/2011/04/Half-done-mocap-retargeting-video
2) http://blog.wolfire.com/2011/04/Half-done-character-skinning-video
3) http://blog.wolfire.com/2011/01/Half-done-character-physics-video
Wow. That is exciting stuff.
*sniff* I wish I had mo-cap.
Well if you need a character animator, I got a lotta free time in the foreseeable future. :P
There’s been a lot of talk about Kinect-based mocap (see iPi Soft for reference). Perhaps it’s time for Christmas-in-July at the Young household, quickly followed by “Shamus writes a cool authoring tool for himself that he then sells”?
Seriously, it could be very worth it as both a time-saving tool (mocap is much quicker than manual animation), and as a potential source of income. There’s a lot of people out there with Kinects, and iPi Soft’s asking price is too rich for my blood.
I think there’s also several OS projects out there using the OS Kinect drivers to do all sorts of stuff. Chances are that by the time Shamus was done writing a Mocap driver for the kinect, there are several Blender games and movies out there using the Blender Kinect mocap module (I’m pretty sure one must be in the works, at least).
I’ve no idea how well suited the blender file format is, but I’m pretty sure there are several tutorials (have seen one or two once) about how to do mo-cap-like animations off of videos in blender.
… oh, I know you’re not a fan of blender, but I’m not sure whether Maya is any better (and if you own it), and since this is “face-your-fears” week anyway, you might want to take a look at the new interface of the current version. It’s supposed to be a lot nicer than the old one*.
Regarding the file format: If you know your way around python, it should also be possible to just ignore blenders file format and have a python script deliver all the necessary data in whatever format you like to have it in.
* I have only casual acquaintance with blender. Meaning to really get into it but alway out of time. So I can’t really give a real recommendation. What I know about the new interface is that it is a lot tidier in many places, so less likely to confuse you. The “each subwindow can be anything” philosophy is still in place, though, and 3D navigation is handled differently by each and every 3D application I know of, anyway. Which is a shame, there really should be some sort of standard.
I hated old Blender and liked new Blender, if that’s worth anything. Like most top-level (“scene”) formats, .blend is not really a good mesh format. It’s designed for flexibility, not performance or ease of parsing – to parse it correctly you actually need to have a parser description parser to read the description that says how to parse everything else! But as I and others have mentioned, writing an exporter is pretty easy if you have an example to work from (and are willing to learn Python, which isn’t as scary as it sounds – it’s the easiest language to learn I know).
Ohh,oooh! Have I linked to this before?
http://www.funkboxing.com/wordpress/?p=267
Very little pre-animated stuff, most just results from programming, and still looks pretty natural.
A similar approach might also work outside of blender and might save you tons of pre-made animation sequences. … and require long hours of writing simulation code, and maybe reading up on kinematics. Not sure, though, if bipeds could work well this way. The ones in the progranimation video look a bit jerky.
Hey, thanks for posting this. I found that project about 6 months ago… and then completely lost track of it… including forgetting the name. Thanks for pointing me back at it.
Yay! Yes, I came across that once before, thanks for bringing it up again.
Especially the “half-done rigging” video is pure gold. Sometimes I wish someone made a game with those Dali-esque animation errors. It looks so beautiful! (And I’m serious, really)
One: I am in awe. I knew something BIG was coming but this is HUGE. Awesome job, Shamus! Two: Paragraph 2, sentence 3 “Two two or three” I think you know what must be done ;-) Three: Your progress is pretty amazing here. The back of my head is starting to expect to be playing this within the next six months.
Also bullet point 1 sentence 3 “Some hard harder to read than others.”
I have nothing else to add besides “This is still cool.”
Basic animation like this is hard enough, but working with character animation in a full-size production is quite possibly of the most difficult and demanding jobs in game development. I’ve had a hand at creating cutscenes for 3D games before and anything more complex than “play animation while standing at X” tends to be extremely challenging and time-consuming. I’ve heard stories from animators saying they had to blend hundreds of animations together over the course of a second or two just to get their desired effects, and I believe them. I also can’t believe they haven’t gone insane and murdered all their co-workers.
I think you want “Some hard harder to read than others” to be “Some are harder to read than others
I think he actually meant “Some hard harder to hard than hard.”
That’s what she said.
http://instantrimshot.com/classic/?sound=rimshot
…
…So, if I have read your post right, rather than fight with the turgid documents of a thousand previous programmers, you are building a character animation and building system from scratch?
Sir, I have just specially put a hat on and tipped it to you.
Hope it all works out.
Well er, he’s still importing from elsewhere. He’s just working from his system to theirs, instead of from theirs to his.
At least, I really hope he’s not making his own 3-D modeler tool. As cool as it would be, we really don’t need that wheel re-invented AGAIN – at best, we want people to contribute to what exists and FIX the problems in the current projects (Updating documentation is something noone wants to do, apparently. Not that I don’t see why – I do test case documentation where I work, and we’ve just upheaved a LOT of functionality – not looking forward to re-writing the test cases to be more accurate…).
My guess is that you can keep the quality of 3d modeling lower, and still produce something in line wiht the quality of the scenery, if you go with an alien or animal rather than a human avatar. Isn’t that what Pixar did in its early movies””focus on animals and toys because they were easier to render convincingly?
I think it’s not so much easier to make realistic, as our expectations being lower. A human should look at least somewhat like an actual human, while animals and toys don’t so much.
In general, humans have a very strong mechanism with sole purpose of identifying fellow, healthy humans.
Because of it, if human characters are animated or drawn just slightly unrealistic – but not unrealistic enough – they tend to make the viewers feel extremly uneasy. Characters that cause such reaction are described as being in ‘uncanny valley’.
You can consult this Manly Guys Doing Manly Things post or these Extra Credits, around 4 minutes mark.
I’m wondering, why don’t you use existing library for the animation ? I’m not an expert but it seems to be a lot of library existing for that, most open source. is it because you want to do everything yourself?
Nope. No libraries for it. Not unless I want to use someone else’s entire graphics engine.
What about Cal3D? Also, have you considered using MD2 as a model format?
What about for the in-game physics?
i heard some good of ode http://www.ode.org/
In my opinion the golden age of ODE is long time gone (supposing it has picked up for real in the first place). ODE might have been the gold standard years ago but it’s a real pain to deal with. Barely no documentation at all, no SDK to start with (unless you consider the source to be an SDK), little to no demos at all…
I suggest to use bullet instead (I suppose that’s why Bob linked it). In a certain sense, it is ODE’ spiritual successor. The documentation is still rough but the SDK includes plenty of demos covering almost everything. Also comes with no-pain-involved build settings. Yes.
MD2 is an *awful* model format. Vertex precision is terrible, and it’s vertex animated rather than skeletal. Bare minimum for a current engine is MD4 (Quake 3 Team Arena).
Of course, a model format is pretty trivial: A attributes (eg: pos xyz, normal xyz, texcoord xy, boneindex) * B vertices for the mesh + C bones * D frames for the animation. I just write the Blender exporter myself, which was surprisingly easy once I found a basic example of an exporter.
You forgot a very important animation that is of vital importance: “pick up poop from loo, throw at wall”.
Great article. I love seeing stuff like this done from the ground up.
Good luck!
Cool stuff. 3D game design is entirely terrifying to me. I think it’s because you have to call so many predefined functions and stuff to get anything done, and I’m very uncomfortable doing things without knowing exactly why they’re working. For instance, I have no idea why redrawing stuff needs to take “Graphics g” as an argument.
“This should also explain the bug you may see in videogames once in a long while: You'll see this brief glimpse of a character standing arms-out, feet slightly spread. That's a character that hasn't had any animation applied.”
I had that happen in Oblivion. The guy was frozen in place, arms out, and being treated as a container. I guess it was a corpse that was never triggered to start being a ragdoll or something.
(Pic: http://i52.tinypic.com/2evt89v.png )
I’ve seen this in various games fairly often, particularly in MMOs when an area is first loading. You often get crowds of players standing like that when the area has loaded, but the PCs haven’t yet.
Shouldn’t it be trival to set the default pose to something that appears more natural? Isn’t it just a set of numbers?
Since you’re building the entire thing from scratch, making no attempt to match existing libraries, why not assign each bone in the skeleton a length and (optionally) range of motion? Then describe each movement as “From (azimuth, elevation, rotation) to (azimuth, elevation, rotation) in (time) rather than needing (x,y,z,top) to (x,y,z,top).
Getting the animations to work properly with scenery is so nontrivial I can’t conceive of a way to do it right without applying full collision detection and simple physics; you might be best off using the quick, dirty, cheating techniques of ramps instead of stairs, letting the feet clip uneven ground, and fudging some (literal) edge cases where one or both feet might not be above the surface supporting the character.
Wasting your time? It’s a blog, this is much more interesting than most posts in people’s blogs. So, what’s the problem?
And I don’t think Shamus is doing Project Frontier with the explicit goal of making money. It may do so, but it is, at the moment, just a very interesting experiment.
Edit: Oh crap, I responded to a deleted post :)
Ah, the joys of working bottom-up instead of top-down. (When you know approximately what you’ll need to do at the bottom levels, anyway, which — having apparently done animation before — you do here.)
It’s actually possible to *debug* the code. :-)
(Well, maybe “bottom-up” and “top-down” aren’t perfect here. But “the joys of being able to work close to the visual system that’s already in place, so you can see that the code is working properly, rather than being forced to assume the code you just wrote is correct and just keep building on it until you have a monstrosity full of points where bugs might lurk” is quite a bit longer.)
Can’t wait to read which graphics format you picked. Every time I’ve looked at it, there’s just a mess of poorly documented formats that no one supports completely.
— edit — never mind, no libraries :)
Most 3D software usually has some kind of plugin architecture doesn’t it? If the documentation for that is less horrible than the file format documentation you could always avoid the latter by using the former to write your own exporter/importer pair. Writing the pair should be much less than 2x the work of just an importer since it’s mostly just swapping out writes for reads and whatnot. I have no idea if the plugin documentation for any given program *is* better enough to go this route, but it’s a possibility at least.
I found Blender pretty easy to write an exporter for. It’s just a python file, no header files or crazy compilation requirements!
Re: The T-Pose stuff
So that’s what happened here…
http://www.youtube.com/watch?v=Da09Wel-gkE&feature=player_detailpage#t=383s
Well…that’s an A-Pose. Actually Characters are more often created in that position instead of T, because it is a more relaxed position for the shoulder and that helps with Rigging and Animation.
He’s such a cute guy! Can we pick a name for him?
eh… Ciao would be nice. ;-P do anyone remember italia 90 mascotte?
worst mascotte ever
I remember him albiet vaguely. Didn’t he have a soccer ball for a head or something like that? I have no idea.
It had it. such a shame
I really like that last shot of the strange, alien stickman wandering around a meadow.
There’s something strangely poignant yet unsettling about the idea of that lone stick-being wandering alone around Shamus’s vast, empty, but beautiful, world.
And for the record, I think ‘he’ is definitely a she!
Programming is debugging, as they say.
Well, if you think the proportions are off, you can always go back to the ancient masters. Still, pretty cool for a first try.
I’ve always found that the best way to build a complex application is by doing things in small increments, so you can see right away when you’ve made a mess, and not two to three fully-implemented modules further ahead… which is exactly what you’re doing. Congratulations, sir.
Well I’m glad your taking precautions to stop the project from dying because that would suck. Hope this whole character model stuff works out.
And yet you still gave us pretty screenshots to look at. Tsk, tsk.
You’ll have to be careful Shamus. If you don’t start getting better at under-delivering (or at least not greatly over-delivering) then we’re all going to start taking you for granted… you know, more than we already do. :)
Every time you introduce something new to us I start reimagining what the finished product will look like. Now this world definitely seems like it just HAS to be populated by 8′ tall 75lb aliens with somewhat pointed faces. And it looks amazing. And of course next post you’ll show something new, or the character animations further fleshed out (pun intended) and the world in my mind gets completely overwritten.
And I’m loving that. Thanks for sharing with us all.
You promised there would be no bling bling screenshots. Only glorious walls of text. I’m disappointed. Sorely disappointed.
(That said, right on, Shamus. I enjoy reading your blog about this project, even if 90% of it is over my head.)
I heartily concur – I took a class in Java in college and promptly decided I would never program anything again, but I find this entire world building project incredibly fascinating.
The Wavatar PHP doesn’t work!
Wow, now I know why the biggest features the FIFA devs are always bragging about are the new animations. And I thought making levels for Portal 2 was hard O_o
And here I thought the biggets hurdle of a FIFA game was the level design.
3. You need animations for standing up from behind the chest-high wall, standing up from crouching over the face of the fallen enemy, and bunny-hopping. The walk animation is optional, since nobody uses it anyway.
After walking and holding a gun, crouching over the faces of my fallen enemies is the thing I do most often.
Did you do any trickier tests than the sine wave limb motion? I’m wondering if there’s potential for the animation system to fall over when doing more complicated than that – for example, when moving across the terrain (i.e. animation while you’re translating the model)?
Hi Shamus, why don’t you make your own file formats that are maximally efficient for your situation? Although writing a compiler/converter from a third party file format to your own takes some extra effort (although I imagine this would be fairly easy), I think it has 2 major advantages:
1) You get to select the third party format purely based on what modeling/animation tools you want to use, or how easy the format is to parse without having to worry about efficiency (both in terms of extra data/memory and parsing speed).
2) In your game you get to use maximally efficient and flexible file formats because you can custom tailor them to your own unique situation.
For your own formats it would probably be enough to just (de)serialize the object that you are using for your model/animation in the code, so that doesn’t really seem like it would be a lot of work to me, but I could be wrong.
Still looking good so far. What kind of software are you looking to use to make the models? God forbid you go back to blender again :(.
Yeah, every now and then in CoH, a soldier goes into that position, his gun hanging in front of him, then he floats around the map, firing bullets from his torso. Good times.
Hmm, I was under the impression that the “T” pose was because if you create a character model “arms down”, then raising the arms will give you horrible texture stretching underneath. The opposite isn’t really a problem, because nobody is going to notice if the texture under a guy’s arms is crunched down. After all, what modelling software doesn’t give you the option of hiding faces you aren’t working on?
(Disclaimer: I’ve never been able to get the hang of character modelling, I’ve only played around with environment/architectural modelling, and not a huge amount of that either)